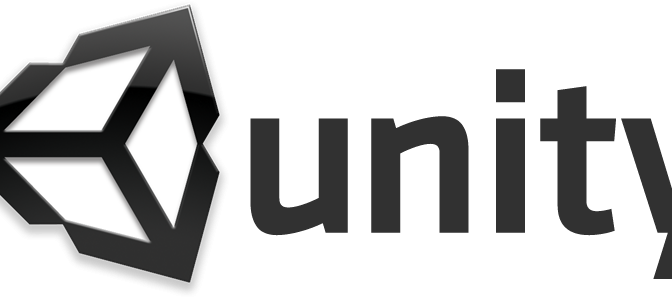
How to reference an array of scripts from another script in Unity
Just a quick and dirty tip (actually, more of a reminder for myself), probably there’s a better way but for now it worked for me… Basically in Unity I needed a quick way to reference a list of scripts from another script. The idea is to have a “main” script holding a list of sub-scripts used to create instances of different game objects (some kind of dynamic factory). The first step is to create a base class for the sub scripts:
[csharp]
using UnityEngine;
public abstract class HazardCreator : MonoBehaviour {
public abstract GameObject CreateHazard ();
}
[/csharp]
then some subclasses:
[csharp]
public class EnemyCreator : HazardCreator {
public override GameObject CreateHazard(){ ….. }
}
public class AsteroidCreator: HazardCreator {
public override GameObject CreateHazard(){ ….. }
}
[/csharp]
then in the game hierarchy I created a GameObject used as “controller” and a child GameObject that contains all the factory scripts. As last step I assigned a script to the “controller” GameObject:
[csharp]
public class GameController : MonoBehaviour {
void Start ()
{
var factories = this.GetComponentsInChildren
[/csharp]
As you may easily notice, the call to GetComponentsInChildren is helping us getting all the scripts inheriting from the HazardCreator base class 🙂