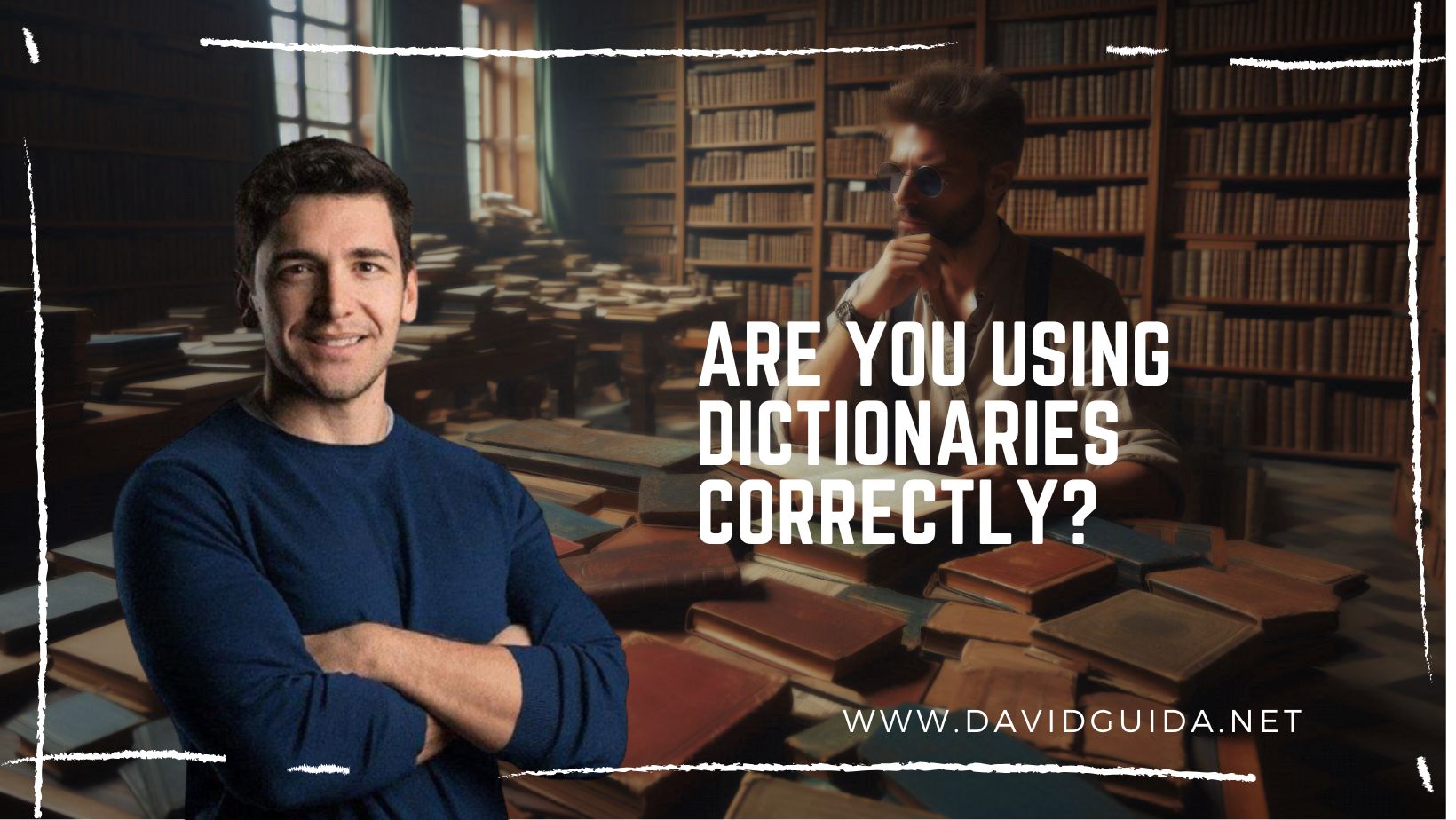
Are you using Dictionaries correctly?
A while ago I wrote a small comparison between Dictionary, HashSet, SortedList and SortedDictionary when performing a lookup operation.
I have noticed however that many developers don’t use the most efficient method for checking if an item exists and retrieving it.
Most of the times, you’ll see something like this:
if (myDictionary.ContainsKey(key))
{
var value = myDictionary[key];
// do something with value
}
…which is fine and does its job. But if this code is in performance-sensitive paths, there’s a better and more concise way:
if (myDictionary.TryGetValue(key, out var value))
{
// do something with value
}
“No big deal”, you might say. Well, this benchmark says otherwise:
The calls to TryGetValue
are almost 2x faster! But why is that?
The reason is pretty simple actually: calling ContainsKey
and then accessing the value by key is performing the lookup twice.
As you can see, I have also included a comparison between .NET 8 and 9. The difference is not abyssal, but we can still see an improvement.
As usual, the code for the benchmark is available on GitHub.
Enjoy!